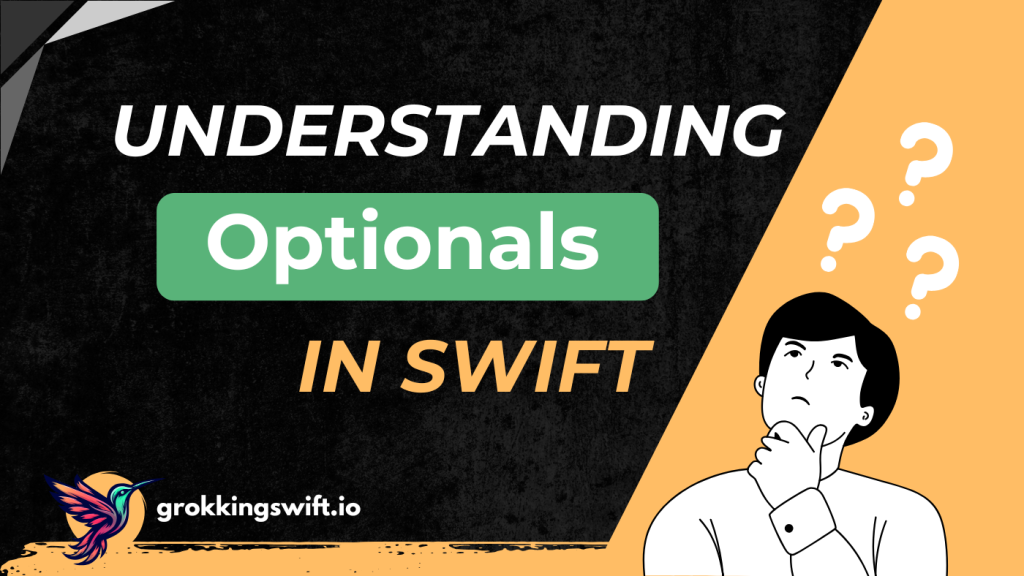
Swift’s introduction of optional types marked a significant advancement in the way developers handle the absence of values, or nil, in their code. This feature is a cornerstone of Swift’s design, aiming to improve code safety and clarity, especially when compared to Objective-C’s approach to nil values.
Design and Internal Mechanism
An optional in Swift is a type that can hold either a value or nil to indicate the absence of a value. They are similar to nullable references in other languages, but with a more explicit and safer approach. Every type in Swift can be made optional by appending a trailing question mark (?) to its type. For example, Int? represents an optional integer which can be an integer or nil. Under the hood, Swift’s optionals are implemented as an enum, which looks like this:
@frozen public enum Optional<Wrapped> : ExpressibleByNilLiteral {
// The absence of a value.
case none
// The presence of a value, stored as `Wrapped`.
case some(Wrapped)
}
So, technically, the following forms of optional type are all perfectly valid:
let numA: Int? = Int("36")
let numB: Optional<Int> = Int("36")
let numC: Int? = Optional.some(36)
let numD: Int? = Optional.none
To access the value inside an optional, you must unwrap it. Swift provides several methods for safely unwrapping optionals:
- Optional Binding (
if let
andguard let
): Safely unwraps the optional if it contains a value, otherwise skips the block of code. - Forced Unwrapping (
!
): Directly accesses the value inside the optional. However, if it’s nil, your app will crash. - Optional Chaining (
?
): Allow the safe calling of properties, methods, and subscripts on an optional that might be nil. - Nil Coalescing Operator (
??
): Provides a default value for an optional if it is nil.
The advantages over Objective-C nil handling
Objective-C allows any object to be nil and sending a message to nil is perfectly valid, returning a zero value. This behavior can lead to bugs that are hard to track down because it’s not always clear where or why nil was introduced.
On the other hand, Swift’s optional types force developers to explicitly handle nil values in their code, making the potential absence of a value clear and explicit. Swift’s compiler performs checks to ensure optionals are handled correctly reducing the chances of nil-related runtime errors.
The Common Use Cases
Using optionals in Swift is a best practice in many scenarios, especially where the presence of a value is uncertain or can change overtime. Let’s examine a few use cases illustrating where optionals are the ideal choice:
1. Handling network requests and responses
When making network requests, the response from the server might not always contain the data you expect. Optionals allow you to safely handle these cases.
func fetchUserProfile(from url: URL, completion: @escaping (UserProfile?) -> Void) {
URLSession.shared.dataTask(with: url) { data, response, error in
guard let data = data, error == nil else {
completion(nil) // Network error or no data
return
}
let userProfile = try? JSONDecoder().decode(UserProfile.self, from: data)
completion(userProfile) // userProfile is nil if decoding fails
}.resume()
}
2. User input that can be optional
User input in forms or dialogs might be optional as the user can leave some fields empty.
class RegistrationForm {
var username: String
var email: String
var phoneNumber: String? // Optional because the user might not provide it
init(username: String, email: String, phoneNumber: String?) {
self.username = username
self.email = email
self.phoneNumber = phoneNumber
}
}
3. Dealing with collections and their elements
When accessing elements in an array or dictionary, the element might not exist for the given key or index, making optionals a safe choice.
let fruits = ["apple", "banana", "cherry"]
let firstFruit = fruits.first // Optional, as the array might be empty
let scores = ["John": 89, "Jane": 92]
let score = scores["Jake"] // Optional, as "Jake" might not have a score
4. Interacting with APIs that can return nil
When interacting with APIs, especially ones that bridge with Objective-C, many return types are optionals because they can return nil.
let image = UIImage(named: "nonexistentImage")
// Returns an optional UIImage because the image might not exist in the bundle
5. Failable initializer
Initializers that might fail to properly instantiate an object, due to invalid input or other conditions, should return an optional instance.
class Product {
var name: String
var price: Double
init?(name: String, price: Double) {
guard price > 0 else { return nil } // Fails initialization if price is not positive
self.name = name
self.price = price
}
}
6. Properties that are set later
Sometimes, properties of a class or struct cannot be set during initialization and need to be optional until a value can be provided.
class ViewController: UIViewController {
var userProfile: UserProfile? // Set after fetching data from a network request
}
Best Practices
As we have discussed earlier, since you have to deal with optionals explicitly, Swift provides several ways to safely unwrap them. Using which approach depends on your specific requirements. However, there are some key guidelines that you can follow to ensure your code is both safe and efficient.
- You should avoid forced unwrapping or implicitly unwrapped optionals except in cases where you’re absolutely sure an optional won’t be nil. Although implicitly unwrapped optionals are useful in certain contexts (like Interface Builder outlets), their overuse can lead to unsafe code.
- Optional binding using
guard let
is preferred for early exits. This makes your code cleaner and avoids deep nesting. - If you need a default value for an optional if it is nil, consider the nil coalescing operator (
??
). - Finally, when designing functions or models, think carefully about which values can be nil and declare them as optionals accordingly.
Conclusion
Optionals in Swift are not merely a feature; they are a fundamental shift towards safer and more expressive code. They force developers to explicitly deal with the absence of values, greatly reducing the common pitfalls associated with nil values in programming. Embracing optionals and understanding their best practices is crucial for any Swift developer aiming to write high-quality, safe Swift code.
Thanks for reading! 🚀